CS 184: COMPUTER GRAPHICS
PREVIOUS
< - - - - > CS
184 HOME < - - - - > CURRENT
< - - - - > NEXT
Lecture #11 -- Mon 2/28/2011.
Warm-up Exercise:
Propose a
good bounding volume hierarchy for ray-tracing this scene:
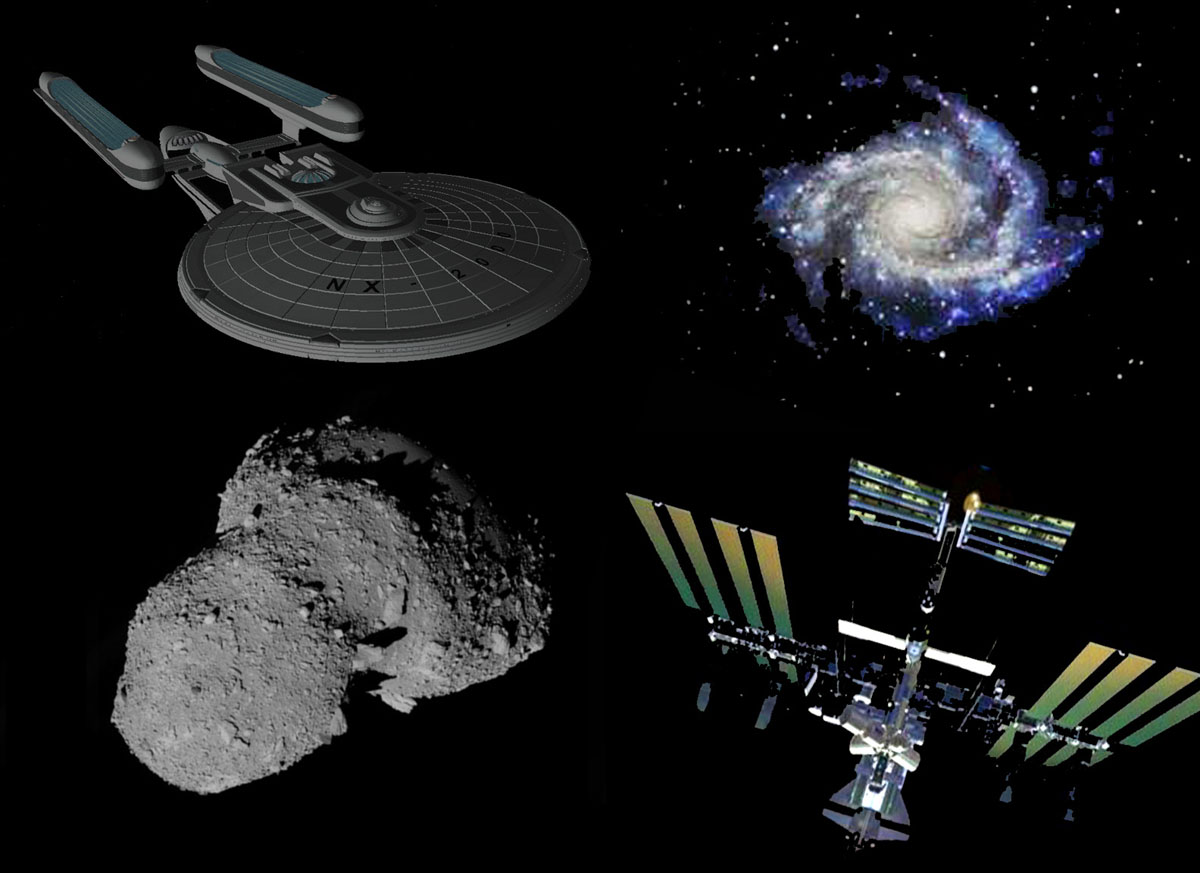
|
In
the foregrounds is a space-station (S) rotating and changing shape; it
has a well structured scene-graph based on its articulated joints and
is composed of a total of 106
triangles. In the middle-ground there
is an irregular heavily pocketed asteroid (A) drifting from bottom to
top across the scene; it is represented by a volumetric density model
comprising 108 voxels; its overall shape is fixed. Also in the
middle-ground is a rocket-ship (R) moving rapidly from upper left to lower right; it is made up of a total of 107 triangles organized in a
5-level deep object description hierarchy. In the background there is
a spiral galaxy (G) composed of a total of 1010 stars represented as
an unstructures sea of point light sources. |
Ray-Tracing (cont.)
Eficiency Aspects: Exploiting Locality and Coherence
Ray - Bbox Intersections [10.9.1] {12.3.1}
Parameterize ray; determine parameter values for when ray crosses xmin, ymin, zmin, xmax, ymax, zmax.
Ray passes through box, if the union of all three inside-intervals [ (xmin,xmax), (ymin,ymax), (zmin,zmax) ] is non-zero.
Hierarchical Bounding Volumes [10.9.2] {12.3.2}
There are many variant for this ...
1.) The AABB's derived from the hierarchy of your scene graph (as in assignment#3).
Example: Rollercoaster with two cars.
2.) Recursive Median Partitioning of the Scene Content (recommended for your Assignment#6):
Instantiate all objects in your logical scene hierarchy -- e.g.,
spheres sprinkled by a generator program,
(or triangle meshes read in as
OBJ files).
Now you have hierarchically flat, fully instantiated "sea of spheres
(and triangles)".
Determine the centroids for each primitive, and the
overall bounding box around the whole scene.
Pick the longest axis of this bounding box, and sort all centroids
along this axis;
then split the whole population at the median into two
halves of equal magnitude.
Recursively continue this splitting process for the two halves.
When you have created the complete binary tree, recursively from the
leaves to the root,
create a nested AABB hierarchy, starting
with the bounding boxes of the leaf-primitives.
After having built this additonal data-access structure,
you can now do
the queries as to what each ray intersects much more efficiently.
==> Compare the rendering times in your ray-tracer with and without using this data structure.
Other methods to create efficient testing for Ray-Scene intersections (which you need to know for future exams):
Flatten scene into a "sea of primitives."
Fill the overall bounding box around the whole scene with a uniform 3D
grid (about a thousand grid cells for a million triangles).
Link each primitive to all the cells that it overlaps.
A ray traverses the scene going from cell to cell (starting from the
eye);
for each cell, test all the primitives linked to it (monitor range
of "t" to keep ray in cell).
Example: Three objects in uniform grid
As for the Median Scene Partitioning scheme, split space
recursively into a balanced binary tree, but now along arbitrary planes!
At each node we keep the equation of the splitting plane
and pointers to the two subtrees on either side of it,
which
contain all objects that touch that space (Objects that straddle the splitting plane will be linked to both subtrees).
A ray first tests the subtree on the side of the plane where it
originates;
if there is no hit, it will test the contents of the other side
of the plane.
[See Shirley Ch. 8.1 for a BSP tree in the context of hidden surface
elimination; (split along the arbitary planes defined by individual polygons)]
Example: Space partitioning on CG-model of Soda Hall
5.) Mixed, Structural and Adaptive Partitioning
The techniques described above can be mixed and combined to best fit a scene of a special type:
For instance, if the scene has a few complicated (group-)objects slowly moving
against one another:
==> separate partitionings could be done withing the
bounding boxes of every (group-)object.
If the density of primitives varies strongly within the whole scene or
within any such object bounding box,
==> then an adaptive
octree-based space partitioning could be used.
Rays interrogate the Bboxes of the logical scene hierarchy as in the scene-based Hierarchical Bounding Box scheme;
then inside the leaf boxes of the scene-tree they proceed in a
spatially ordered manner as in one of the space partitioning schemes.
Example: Warm-up question above
Geometrical Aspect: Ray - Triangle Intersections [10.3.2] {4.4.2}
Ray equation: R(t) = A+tD
Triangle in barycentric coordinates: X(β, γ) = V1+β(V2−V1)+γ(V3−V1)
Combine to find intersection point: V1+β(V2−V1)+γ(V3−V1) = A+tD
Solve
for β, γ, and t: ==> 3 equations (in x, y, z) with 3
unknowns; ==> danger: divide by near-zero; ==> check ranges.
Knowing β and γ also allows for easy (linear) interpolation of any vlaues (e.g., color) assigned to the vertices.
(Triangles and triangle meshes are an extra-credit extension on assignment #6.)
Ray-tracing versus the Classical Rendering Pipeline
Ray-tracing gives highest quality pictures; ==> used in CG movies.
Classical pipeline gives higher performance; ==> used in video games.
Discussion of Take-Home Exam (cont.)
Min=37, Max=108, Mean=78, Median=80, Stdev=16; Approximate evaluation: A=95; B= 80; C=65.
If you find any grading problems, please describe the problem on the back of the exam, or on a separate sheet of paper,
and hand the exam back to me; we will then take a second look at that problem.
Next lecture:
How Can We Describe 3D Objects ?
Voxels
and Octree:
Concept: sample space regularly;
determine whether the sample falls inside or outside of object, e.g., by an implicit function;
"turn on" inside voxels.
Efficient encoding required: e.g., quadtree in 2D, octree in 3D.
Good for representing: MRI-scans, CAT-scans, brains, sponge-like objects,
clouds, ...
CSG
and Boolean set operations:
CSG = Constructive Solid Geometry.
The object is composed from (overlaping) primitive geometric shapes,
typically: cubes, halfspaces, spheres, cylinders, cones, tori, ...
e.g., a "Sausage" = bent cylinder plus spherical end-caps (show 2D
composite).
Good for representing: mechanical parts, Swiss cheese, results of solid
modeling operations, ...
B-rep
and winged-edge data structure:
Tessallate surface; describe polyhedral objects via vertices, edges, faces;
or "meshes" (triangle strips, fans) that use a more efficient encoding
of the connectivity.
Good for representing: polyhedral objects with odd angles, tessellated
parametric surfaces, ...
Procedural modeling:
A program fragment creates the vertices and faces and their connectivity,
or, alternatively, the instance calls to CSG primitives,
or a collection of "inside" voxels,
or an implicit function, which may then be converted to one of the
above representations.
Good for representing: parameterized, iterated objects;
e.g., gearwheels, fractal mountains, trees, geometrical
sculpture, ...
Commonly used generic procedures: Sweeps:
(axial, rotational, or along an arbitrary path).
For "Sausage": Sweep a circle through space along a curve. Start small
for front-cap;
keep constant through main part of sausage; taper down to zero for
end-cap.
Good for representing: pipes, sausages, moldings, rotationally symmetrical
(turned) objects, geometrical
sculpture, ...
Reading Assignments:
Study: ( i.e., try to understand fully, so that you can answer
questions
on an exam):
Shirley: [ 2nd Ed: Ch 8.1, 10.10, 13.1-13.2]
Shirley: { 3rd Ed: Ch 12.1, 12.3-12.5}
Programming Assignment #5: To be done individually; due (electronically submitted) before Saturday 2/26, 11:00pm.
Programming Assignment #6: May be done in pairs; due (electronically submitted) before Saturday 3/5, 11:00pm.
PREVIOUS
< - - - - > CS
184 HOME < - - - - > CURRENT
< - - - - > NEXT
Page Editor: Carlo
H. Séquin